Fundamentals of Python Interview Questions
- Home
- »
- Fundamentals of Python Interview Questions
- What is Power BI?
- What is Power BI Desktop?
- How is Power BI Desktop different from Power BI Service?
- What are the main components of the Power BI Desktop interface?
- What is the Fields pane in Power BI Desktop used for?
- How can you connect Power BI Desktop to data sources?
- What is Power BI Mobile?
- How can you share Power BI reports with others using the Power BI Service?
- What is Power BI Mobile?
- How can you integrate Power BI with other Microsoft tools and services?
- How can you zoom in and out on a Tableau worksheet?
- What is the purpose of establishing relationships between tables in Power BI?
- How can you create relationships between tables in Power BI?
- What are the different types of relationships supported in Power BI?
- How can you enhance data modeling by creating calculated columns in Power BI?
- What is a measure in Power BI?
- How can you create a measure in Power BI?
- Can you create hierarchies in Power BI? If so, how?
- How can you enhance data modeling by using calculated tables in Power BI?
- What is the role of data modeling in Power BI report performance?
- What is data visualization in Power BI?
- What are the different types of visualizations available in Power BI?
- How can you create a visualization in Power BI Desktop?
- How can you enhance visualizations in Power BI by adding filters and slicers?
- What is a drill-through in Power BI?
- How can you create a dashboard in Power BI?
- Can you add custom visuals to Power BI reports and dashboards? If so, how?
- How can you enhance data visualization in Power BI by applying filters and highlighting?
- What is the role of drill-through and cross-filtering in Power BI dashboards?
- How can you share Power BI reports and dashboards with others?
- What is DAX in Power BI?
- How can you create a calculated column using DAX in Power BI?
- What is the difference between calculated columns and measures in Power BI DAX?
- How can you use DAX to filter data in Power BI?
- What is the purpose of the aggregation operator (SUM) in Tableau?
- How can you use DAX to perform time intelligence calculations in Power BI?
- How can you use DAX to perform conditional calculations in Power BI?
- What is the role of the CALCULATE function in DAX, and how is it used?
- How can you use DAX to calculate running totals in Power BI?
- How can you optimize DAX calculations for better performance in Power BI?
- What is Power Query in Power BI?
- How can you access Power Query in Power BI?
- What are the benefits of using Power Query in Power BI?
- How can you combine multiple data sources using Power Query in Power BI?
- What is data cleansing, and how can you perform it using Power Query?
- How can you split columns using Power Query in Power BI?
- Can you create custom columns using Power Query in Power BI? If so, how?
- How can you transform and clean data using Power Query functions?
- How can you handle null or missing values in Power Query?
- Can you create reusable query steps in Power Query? If so, how?
What is a variable in Python?
Answer: A variable in Python is a named identifier that holds a value. It allows you to store and manipulate data in memory.
How do you declare a variable in Python?
Answer: In Python, you can declare a variable by assigning a value to it using the assignment operator (=). For example, x = 5 declares a variable named x and assigns it the value of 5.
What are the rules for naming variables in Python?
Answer: Variables in Python must follow certain rules:
They can contain letters, numbers, and underscores.
They cannot start with a number.
They are case-sensitive.
They should have descriptive names to enhance code readability.
What are the different data types in Python?
Answer: Python has several built-in data types, including:
Numeric types: int, float, complex
Sequence types: list, tuple, range
Text type: str
Mapping type: dict
Set types: set, frozenset
Boolean type: bool
How can you determine the data type of a variable in Python?
Answer: You can use the type() function to determine the data type of a variable in Python. For example, type(x) returns the data type of the variable x.
What is the difference between integers (int) and floating-point numbers (float) in Python?
Answer: Python provides built-in functions for data type conversion, such as int(), float(), str(), and bool(). These functions allow you to convert variables from one data type to another.
What is the difference between integers (int) and floating-point numbers (float) in Python?
Answer: Integers represent whole numbers without decimal points, while floating-point numbers represent numbers with decimal points. Floats can store larger and more precise numbers, but they require more memory compared to integers.
How do you format strings in Python?
Answer: You can format strings in Python using the format() method or f-strings (formatted string literals). Both methods allow you to insert variable values into strings and control the formatting of the output.
How do you check if a variable belongs to a specific data type in Python?
Answer: You can use the isinstance() function to check if a variable belongs to a specific data type. For example, isinstance(x, int) returns True if the variable x is an integer.
How can you perform arithmetic operations with different data types in Python?
Answer: Python supports arithmetic operations between compatible data types. For example, you can perform addition, subtraction, multiplication, and division on integers and floats. However, operations between incompatible data types may result in errors.
Operators
What is an operator in Python?
Answer: An operator in Python is a symbol or keyword that performs a specific operation on one or more operands to produce a result.
What are the different types of operators in Python?
Answer: Python has several types of operators, including:
Arithmetic operators: +, -, *, /, %, **
Assignment operators: =, +=, -=, *=, /=
Comparison operators: ==, !=, >, <, >=, <=
Logical operators: and, or, not
Bitwise operators: &, |, ^, ~, <<, >>
Membership operators: in, not in
Identity operators: is, is not
What is the difference between the '==' operator and the 'is' operator in Python?
Answer: The ‘==’ operator compares the values of two objects, while the ‘is’ operator compares the identity of two objects. ‘==’ checks for equality, whereas ‘is’ checks if two objects refer to the same memory location.
How do you perform exponentiation using the power operator in Python?
Answer: The power operator in Python is ‘**’. For example, 2 ** 3 calculates 2 raised to the power of 3, resulting in 8.
How can you concatenate two strings using the '+' operator in Python?
Answer: You can concatenate two strings using the ‘+’ operator. For example, ‘Hello’ + ‘World’ results in ‘HelloWorld’.
What is the result of the expression 10 // 3 in Python?
Answer: The ‘//’ operator performs floor division and returns the quotient without the remainder. Therefore, 10 // 3 evaluates to 3.
How can you check if an element is present in a list using the 'in' operator in Python?
Answer: The ‘in’ operator can be used to check if an element is present in a list. For example, ‘apple’ in [‘apple’, ‘banana’, ‘orange’] returns True.
What is the result of the expression True and False in Python?
Answer: The ‘and’ operator returns True if both operands are True. Therefore, True and False evaluates to False.
How can you perform a left shift operation using the '<<' operator in Python?
Answer: The ‘<<‘ operator performs a left shift operation, shifting the bits of a number to the left. For example, 5 << 2 shifts the bits of 5 two positions to the left, resulting in 20.
How do you negate a boolean value using the 'not' operator in Python?
Answer: The ‘not’ operator negates a boolean value. For example, not True evaluates to False, and not False evaluates to True.
Loops and Conditionals
What is the purpose of loops and conditionals in programming?
Answer: Loops and conditionals allow programmers to control the flow of execution in a program. They enable the execution of certain code blocks based on specified conditions or for a specified number of iterations.
What is the difference between 'if' and 'elif' statements in Python?
Answer: The ‘if’ statement is used to check a single condition, and if it evaluates to true, the corresponding block of code is executed. ‘elif’ is short for “else if” and allows you to check multiple conditions sequentially after the initial ‘if’ statement. If any of the conditions are true, the corresponding block of code is executed, and the remaining conditions are not evaluated.
How does the 'while' loop work in Python?
Answer: The ‘while’ loop repeatedly executes a block of code as long as a given condition is true. It first checks the condition, and if it is true, the code block is executed. Afterward, the condition is checked again, and if it is still true, the code block is executed again. This process continues until the condition becomes false.
What is the purpose of the 'else' statement in a loop?
Answer: The ‘else’ statement in a loop is executed when the loop has completed all iterations without encountering a ‘break’ statement. It allows you to specify a block of code that should be executed after the loop has finished its iterations.
How do you break out of a nested loop in Python?
Answer: To break out of a nested loop, you can use the ‘break’ statement. When encountered, it terminates the innermost loop and continues with the next statement after the loop.
What is the purpose of the 'continue' statement in a loop?
Answer: The ‘continue’ statement is used to skip the remaining code in a loop iteration and move on to the next iteration. It allows you to bypass certain code blocks within a loop based on specific conditions.
What is an infinite loop, and how can you avoid it?
Answer: An infinite loop is a loop that continues executing indefinitely. It can occur when the loop’s condition is always true or when there is no mechanism to terminate the loop. To avoid an infinite loop, ensure that the loop condition can become false at some point or include an exit condition within the loop.
What are nested conditionals in Python?
Answer: Nested conditionals refer to the use of ‘if’ statements inside other ‘if’ statements. It allows you to check multiple conditions sequentially and execute different code blocks based on the outcome of each condition.
What is short-circuit evaluation in Python?
Answer: Short-circuit evaluation is a behavior in Python where the second operand of a logical ‘and’ or ‘or’ expression is not evaluated if the result can be determined based on the value of the first operand. This can optimize the execution of code by avoiding unnecessary evaluations.
What is the purpose of the 'pass' statement in Python?
Answer: The ‘pass’ statement is a placeholder statement in Python that does nothing. It is used when a statement is required syntactically but no code needs to be executed. It is commonly used as a placeholder for future code implementation.
Strings and Regular Expressions
What is a string in Python?
Answer: In Python, a string is a sequence of characters enclosed within single quotes (‘ ‘) or double quotes (” “). It is a built-in data type used to represent and manipulate text.
How do you concatenate two strings in Python?
Answer: Strings can be concatenated using the ‘+’ operator. For example, if ‘str1’ and ‘str2’ are two strings, ‘str1 + str2’ will concatenate them.
What is string interpolation in Python?
Answer: String interpolation is a way to embed expressions or variables within a string to create a formatted string. In Python, it can be achieved using f-strings (formatted string literals) or the ‘format()’ method.
How do you access individual characters of a string in Python?
Answer: Individual characters of a string can be accessed using indexing. Each character in a string has an index starting from 0. For example, ‘my_string[0]’ will give the first character of ‘my_string’.
What is the difference between a mutable and an immutable string in Python?
Answer: In Python, strings are immutable, which means that their values cannot be changed after they are created. However, new strings can be created by manipulating existing strings.
What are escape characters in Python strings?
Answer: Escape characters are special characters preceded by a backslash (”) in a string. They are used to represent characters that are difficult to type or are reserved for special purposes, such as newline (‘\n’) or tab (‘\t’).
What is a regular expression?
Answer: A regular expression is a sequence of characters that forms a search pattern. It is a powerful tool for pattern matching and manipulation of strings. Python provides the ‘re’ module for working with regular expressions.
How do you search for a pattern in a string using regular expressions in Python?
Answer: The ‘re.search()’ function is used to search for a specific pattern within a string. It returns a match object if a match is found, or ‘None’ if no match is found.
What is the purpose of the 're.sub()' function in Python?
Answer: The ‘re.sub()’ function is used to search for a pattern in a string and replace it with a specified string. It replaces all occurrences of the pattern with the replacement string.
How can you split a string into a list of substrings in Python?
Answer: The ‘split()’ method is used to split a string into a list of substrings based on a specified delimiter. By default, it splits the string at whitespace characters, but a custom delimiter can also be specified.
Date and Time Concepts
What is the datetime module in Python?
Answer: The datetime module is a built-in Python module that provides classes and functions for working with dates, times, and time intervals.
What is the difference between 'datetime' and 'date' in Python?
Answer: The ‘datetime’ class represents a specific date and time, including the year, month, day, hour, minute, second, and microsecond. The ‘date’ class represents a date without the time components.
How do you create a datetime object with a specific date and time in Python?
Answer: You can create a datetime object using the ‘datetime’ class constructor and providing the year, month, day, hour, minute, second, and microsecond values as arguments.
How can you get the current date and time in Python?
Answer: You can use the ‘datetime’ module’s ‘datetime.now()’ function to retrieve the current date and time as a datetime object.
What is a timedelta object in Python?
Answer: A timedelta object represents a duration or difference between two dates or times. It can be used for arithmetic operations, such as adding or subtracting time intervals from datetime objects.
How do you format a datetime object as a string in Python?
Answer: You can use the ‘strftime()’ method of a datetime object to format it as a string based on a specified format string. The format string contains special placeholders that represent different components of the datetime object.
What is the UNIX timestamp in Python?
Answer: The UNIX timestamp is a way to represent dates and times as a single number, which is the number of seconds elapsed since January 1, 1970, at 00:00:00 UTC.
How can you convert a string to a datetime object in Python?
Answer: You can use the ‘strptime()’ method of the datetime class to convert a string representation of a date and time to a datetime object. You need to provide the string and a format string that matches the string’s format.
How do you calculate the difference between two datetime objects in Python?
Answer: You can subtract one datetime object from another to calculate the difference. The result will be a timedelta object representing the time difference between the two dates or times.
What is the purpose of the 'time' module in Python?
Answer: The ‘time’ module provides functions for working with time-related operations, such as retrieving the current time, measuring time intervals, and converting between different time representations.
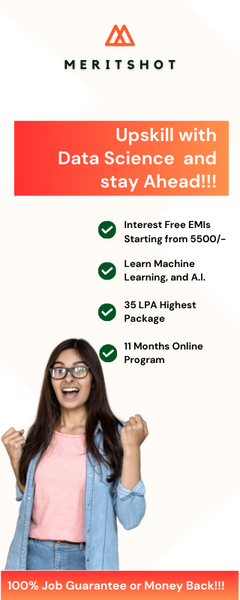