R Programming Language Interview Questions
- Home
- »
- R programming language Interview Questions
- What is R programming language?
- What are the key features of R programming language?
- What is the difference between R and other programming languages like Python or C++?
- How do you install packages in R?
- What is a data frame in R?
- How do you read data from a CSV file in R?
- What is the purpose of the summary() function in R?
- What is the purpose of the apply() function in R?
- How do you handle missing values in R?
- What is the difference between the assignment operator (<-) and the equals operator (=) in R?
- What is vectorization in R?
- How do you create a scatter plot in R?
- What is the purpose of the %in% operator in R?
- How do you generate random numbers in R?
- What is the purpose of the grep() function in R?
- How do you calculate the mean of a vector in R?
- What is the purpose of the aggregate() function in R?
- How do you rename columns in a data frame in R?
- What is the purpose of the unique() function in R?
- How do you check if a value is NA in R?
- What is the purpose of the cor() function in R?
- What is the purpose of the ifelse() function in R?
- How do you create a boxplot in R?
- What is the purpose of the paste() function in R?
- What is the purpose of the glm() function in R?
- How do you check if a value is in a vector in R?
- What is the purpose of the tapply() function in R?
- How do you calculate the mode of a vector in R?
- What is the purpose of the which() function in R?
- How do you create a histogram in R?
What is R programming language?
Answer: R is a programming language and software environment primarily used for statistical computing and graphics. It provides a wide range of statistical and graphical techniques and is highly extensible through packages.
What are the key features of R programming language?
Answer: Key features of R programming language include its ability to handle data manipulation, data analysis, and data visualization tasks. It has a vast collection of packages and functions for statistical modeling, machine learning, and graphics.
What is the difference between R and other programming languages like Python or C++?
Answer: Unlike Python or C++, R is specifically designed for statistical analysis and data visualization. It has built-in functions and packages that cater to these tasks, making it easier to perform statistical operations and generate graphical outputs.
How do you install packages in R?
Answer: Packages can be installed in R using the install.packages() function. For example, to install the “dplyr” package, you can use the command install.packages(“dplyr”).
What is a data frame in R?
Answer: A data frame in R is a two-dimensional data structure that stores data in rows and columns. It is similar to a table in a relational database and is one of the most commonly used data structures in R.
How do you read data from a CSV file in R?
Answer: Data from a CSV file can be read in R using the read.csv() function. For example, to read data from a file named “data.csv”, you can use the command data <- read.csv(“data.csv”).
What is the purpose of the summary() function in R?
Answer: The summary() function in R provides a summary of the statistical properties of a given object, such as a data frame. It gives information like minimum, maximum, quartiles, mean, and count for each variable in the data frame.
What is the purpose of the apply() function in R?
Answer: The apply() function in R is used to apply a function to either each row or each column of a matrix or data frame. It is a convenient way to perform calculations or operations across multiple rows or columns.
How do you handle missing values in R?
Answer: Missing values in R can be handled using functions like is.na() to check for missing values, na.omit() to remove rows with missing values, and na.fill() to replace missing values with a specific value.
What is the difference between the assignment operator (<-) and the equals operator (=) in R?
Answer: In R, the assignment operator (<-) and the equals operator (=) are used interchangeably to assign values to variables. However, the convention in R is to use the assignment operator (<-) for assignments to improve readability.
What is vectorization in R?
Answer: Vectorization in R is the process of applying operations or functions to entire vectors or arrays at once, rather than looping over individual elements. It is a key feature of R that helps in writing efficient and concise code.
How do you create a scatter plot in R?
Answer: A scatter plot can be created in R using the plot() function. For example, to create a scatter plot of two variables x and y, you can use the command plot(x, y).
What is the purpose of the %in% operator in R?
Answer: The %in% operator in R is used to check if elements of one vector are present in another vector. It returns a logical vector indicating whether each element of the first vector is found in the second vector.
How do you generate random numbers in R?
Answer: Random numbers can be generated in R using the runif() function for generating uniform random numbers, the rnorm() function for generating normal random numbers, and the sample() function for sampling from a vector.
What is the purpose of the grep() function in R?
Answer: The grep() function in R is used for pattern matching and searching for specific patterns within character strings. It returns the indices of elements that match the specified pattern.
How do you calculate the mean of a vector in R?
Answer: The mean of a vector in R can be calculated using the mean() function. For example, to calculate the mean of a vector x, you can use the command mean(x).
What is the purpose of the aggregate() function in R?
Answer: The aggregate() function in R is used to compute summary statistics for subsets of data. It allows you to group data by one or more variables and apply a function to each group.
How do you rename columns in a data frame in R?
Answer: Columns in a data frame can be renamed in R using the colnames() function. For example, to rename the first column of a data frame df to “new_name”, you can use the command colnames(df)[1] <- “new_name”.
What is the purpose of the merge() function in R?
Answer: The merge() function in R is used to combine two or more data frames based on a common variable. It performs database-style merging by matching values in the specified variables.
How do you generate a sequence of numbers in R?
Answer: A sequence of numbers can be generated in R using the seq() function. For example, to generate a sequence from 1 to 10 with a step of 2, you can use the command seq(1, 10, by = 2).
What is the purpose of the table() function in R?
Answer: The table() function in R is used to create a contingency table that shows the frequency distribution of categorical variables. It tabulates the counts of each unique value in a variable or combination of variables.
How do you calculate the median of a vector in R?
Answer: The median of a vector in R can be calculated using the median() function. For example, to calculate the median of a vector x, you can use the command median(x).
What is the purpose of the rep() function in R?
Answer: The rep() function in R is used to create a vector by repeating elements. It takes the values to repeat and the number of times to repeat them as arguments.
How do you subset a data frame in R?
Answer: A data frame can be subsetted in R using square brackets [ ]. For example, to subset a data frame df based on a condition, you can use the command subset_df <- df[condition, ].
What is the purpose of the unique() function in R?
Answer: The unique() function in R is used to extract the unique elements from a vector or data frame column. It returns a vector or data frame with only the unique values.
How do you check if a value is NA in R?
Answer: In R, the is.na() function is used to check if a value is NA. It returns a logical vector of the same length as the input, with TRUE indicating NA values.
What is the purpose of the cor() function in R?
Answer: The standard deviation of a vector in R can be calculated using the sd() function. For example, to calculate the standard deviation of a vector x, you can use the command sd(x).
What is the purpose of the ifelse() function in R?
Answer: The ifelse() function in R is used to perform conditional operations. It evaluates a condition and returns values based on whether the condition is true or false.
How do you create a boxplot in R?
Answer: A boxplot can be created in R using the boxplot() function. For example, to create a boxplot of a numeric variable x, you can use the command boxplot(x).
What is the purpose of the paste() function in R?
Answer: The factorial of a number can be calculated in R using the factorial() function. For example, to calculate the factorial of a number n, you can use the command factorial(n).
What is the purpose of the glm() function in R?
Answer: The glm() function in R is used to fit generalized linear models. It allows you to specify various types of response distributions and link functions for different types of regression analyses.
How do you check if a value is in a vector in R?
Answer: In R, the %in% operator is used to check if a value is present in a vector. It returns a logical vector indicating whether each element of the first vector is found in the second vector.
What is the purpose of the tapply() function in R?
Answer: The tapply() function in R is used to apply a function to subsets of a vector or data frame split by one or more factors. It is commonly used to calculate summary statistics by groups.
How do you calculate the mode of a vector in R?
Answer: The mode of a vector in R can be calculated using the table() function to count the frequency of each value and then extracting the value with the highest frequency.
What is the purpose of the which() function in R?
Answer: The which() function in R is used to identify the indices or positions of elements that satisfy a given condition. It returns a vector of indices where the condition is true.
How do you create a histogram in R?
Answer: A histogram can be created in R using the hist() function. For example, to create a histogram of a numeric variable x, you can use the command hist(x).
What is the purpose of the aggregate() function in R?
Answer: The aggregate() function in R is used to compute summary statistics for subsets of data. It allows you to group data by one or more variables and apply a function to each group.
How do you calculate the mode of a vector in R?
Answer: The mode of a vector in R can be calculated using the table() function to count the frequency of each value and then extracting the value with the highest frequency.
What is the purpose of the which() function in R?
Answer: The which() function in R is used to identify the indices or positions of elements that satisfy a given condition. It returns a vector of indices where the condition is true.
How do you create a histogram in R?
Answer: A histogram can be created in R using the hist() function. For example, to create a histogram of a numeric variable x, you can use the command hist(x).
What is the purpose of the boxplot() function in R?
Answer: The cumulative sum of a vector in R can be calculated using the cumsum() function. For example, to calculate the cumulative sum of a vector x, you can use the command cumsum(x).
What is the purpose of the split() function in R?
Answer: The split() function in R is used to split a data object, such as a vector or data frame, into subsets based on one or more factors or variables. It returns a list of data subsets.
How do you calculate the p-value in R?
Answer: The p-value in R can be calculated using various statistical functions and tests such as the t-test, chi-square test, or correlation test. The specific function used depends on the hypothesis being tested.
What is the purpose of the unique() function in R?
Answer: The unique() function in R is used to extract the unique elements from a vector or data frame column. It returns a vector or data frame with only the unique values.
How do you check if a value is NA in R?
Answer: In R, the is.na() function is used to check if a value is NA. It returns a logical vector of the same length as the input, with TRUE indicating NA values.
What is the purpose of the cor() function in R?
Answer: The cor() function in R is used to calculate the correlation between two or more variables. It returns a correlation matrix or a single correlation coefficient, depending on the input.
How do you calculate the standard deviation of a vector in R?
Answer: The standard deviation of a vector in R can be calculated using the sd() function. For example, to calculate the standard deviation of a vector x, you can use the command sd(x).
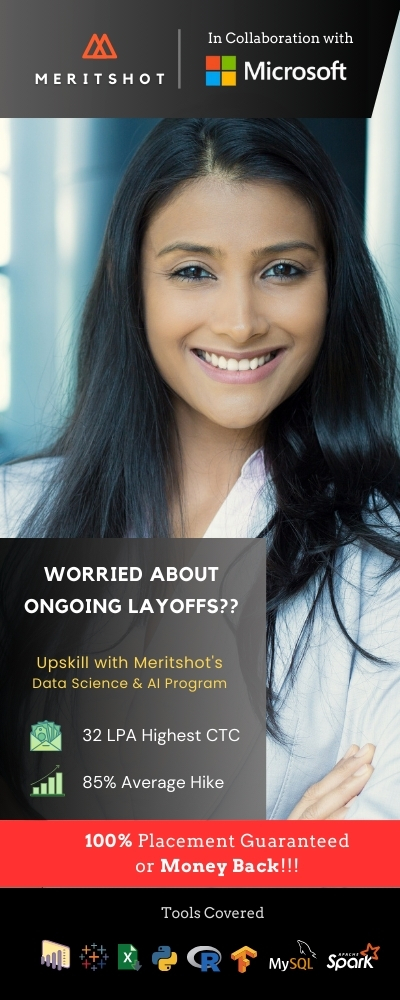