Meritshot Tutorials
- Home
- »
- Serving JSON Responses
Flask Tutorial
-
Introduction to Flask for Machine LearningIntroduction to Flask for Machine Learning
-
Why Use Flask to Deploy ML Models?Why Use Flask to Deploy ML Models?
-
Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)Flask vs. Other Deployment Tools (FastAPI, Django, Streamlit)
-
Setting Up the EnvironmentSetting Up the Environment
-
Basics of FlaskBasics of Flask
-
Flask Application StructureFlask Application Structure
-
Running the Development ServerRunning the Development Server
-
Debug ModeDebug Mode
-
Preparing Machine Learning Models for DeploymentPreparing Machine Learning Models for Deployment
-
Saving the Trained ModelSaving the Trained Model
-
Loading the Saved Model in PythonLoading the Saved Model in Python
-
Understanding Routes and EndpointsUnderstanding Routes and Endpoints
-
Setting Up API Endpoints for PredictionSetting Up API Endpoints for Prediction
-
Flask Templates and Jinja2 BasicsFlask Templates and Jinja2 Basics
-
Creating a Simple HTML Form for User InputCreating a Simple HTML Form for User Input
-
Connecting the Frontend to the BackendConnecting the Frontend to the Backend
-
Handling Requests and ResponsesHandling Requests and Responses
-
Accepting User Input for PredictionsAccepting User Input for Predictions
-
Returning Predictions as JSON or HTMLReturning Predictions as JSON or HTML
-
Deploying a Pretrained Model with FlaskDeploying a Pretrained Model with Flask
-
Example: Deploying a TensorFlow/Keras ModelExample: Deploying a TensorFlow/Keras Model
-
Example: Deploying a PyTorch ModelExample: Deploying a PyTorch Model
-
Flask and RESTful APIs for MLFlask and RESTful APIs for ML
-
Serving JSON ResponsesServing JSON Responses
-
Testing API Endpoints with PostmanTesting API Endpoints with Postman
-
Handling Real-World ScenariosHandling Real-World Scenarios
-
Scaling ML Model Predictions for Large InputsScaling ML Model Predictions for Large Inputs
-
Batch Predictions vs. Single PredictionsBatch Predictions vs. Single Predictions
-
Adding Authentication and SecurityAdding Authentication and Security
-
Adding API Authentication (Token-Based)Adding API Authentication (Token-Based)
-
Protecting Sensitive DataProtecting Sensitive Data
-
Deploying Flask ApplicationsDeploying Flask Applications
-
Deploying on HerokuDeploying on Heroku
-
Deploying on AWS, GCP, or AzureDeploying on AWS, GCP, or Azure
-
Containerizing Flask Apps with DockerContainerizing Flask Apps with Docker
Flask and RESTful APIs for ML
8.1 Building a REST API for Predictions
In this section, we will learn how to build a RESTful API using Flask to serve machine learning (ML) model predictions. A RESTful API allows users to interact with your ML model over HTTP, making it easy to integrate your model with other applications, including web or mobile applications.
We will go through the process of setting up the Flask application to handle HTTP requests, define RESTful routes, and return predictions in a structured format such as JSON.
What is a REST API?
A REST API (Representational State Transfer API) is an architectural style for designing networked applications. It uses HTTP requests to perform CRUD operations (Create, Read, Update, Delete) on resources. For machine learning models, we typically create GET and POST methods for receiving inputs and providing outputs.
In our case, the resource will be the model prediction, and users will be able to interact with the model by sending input data to the API and receiving a response with predictions.
Steps to Build a REST API for Predictions Using Flask
- Set up the Flask Application
First, let’s create a simple Flask application that will expose a RESTful API endpoint for predictions. We will assume the model is already trained and saved.
Flask Application Setup:
from flask import Flask, request, jsonify
import torch
from torchvision import models, transforms
from PIL import Image
import io
app = Flask(__name__)
# Load the pre-trained PyTorch model (replace with your trained model)
model = models.resnet18(pretrained=True) # Example model
model.eval() # Set to evaluation mode for inference
# Define image transformation for input
transform = transforms.Compose([
transforms.Resize(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
@app.route(‘/predict’, methods=[‘POST’])
def predict():
try:
# Get image file from the request
img_file = request.files[‘image’]
img = Image.open(io.BytesIO(img_file.read()))
# Preprocess the image
img_tensor = transform(img).unsqueeze(0)
# Make prediction
with torch.no_grad():
outputs = model(img_tensor)
_, predicted_class = torch.max(outputs, 1)
# Return the prediction in JSON format
return jsonify({‘predicted_class’: int(predicted_class.item())})
except Exception as e:
return jsonify({‘error’: str(e)}), 400
if __name__ == “__main__”:
app.run(debug=True)
In this Flask app:
- /predict endpoint: Accepts a POST request where an image is sent via the request body, and the server responds with the predicted class of the image.
- Image Processing: The image is processed to fit the input format expected by the PyTorch model.
- Prediction: The model performs inference on the processed image, and the class with the highest probability is returned.
Explanation of Code:
- Flask Setup: We start by setting up a basic Flask application and importing the necessary libraries (torch, PIL, transform).
- Model Loading: In this example, we use a pre-trained ResNet18 model from torchvision.models. This can be replaced with your own pre-trained model.
- Image Transformation: The transform variable holds a series of transformations that are applied to the input image to ensure it is correctly formatted for the model.
- /predict Endpoint: The route /predict accepts POST requests with an image file, which is processed and passed to the model for prediction. The result is returned in a JSON response.
Serving More Complex Responses
In addition to simple predictions, your model may return more detailed responses, such as:
- Probability scores: If the model returns probability distributions for multiple classes (such as in classification problems), you may want to return those probabilities.
- Multiple output values: For regression tasks or models with multiple outputs, you can return more than one value in the JSON response.
Here’s an example of returning both the predicted class and the associated probability score:
@app.route(‘/predict’, methods=[‘POST’])
def predict():
try:
# Get image file from the request
img_file = request.files[‘image’]
img = Image.open(io.BytesIO(img_file.read()))
# Preprocess the image
img_tensor = transform(img).unsqueeze(0)
# Make prediction
with torch.no_grad():
outputs = model(img_tensor)
probs = torch.nn.functional.softmax(outputs, dim=1)
predicted_class = torch.argmax(probs, dim=1)
predicted_prob = probs[0, predicted_class.item()].item()
# Return prediction and probability as JSON
response = {
‘prediction’: int(predicted_class.item()), # Predicted class
‘probability’: round(predicted_prob, 4), # Probability of the prediction
‘message’: ‘Prediction successful’
}
return jsonify(response)
except Exception as e:
error_response = {
‘error’: str(e),
‘message’: ‘Error processing the image’
}
return jsonify(error_response), 400
In this example:
- The model returns the predicted class as well as the probability score for that class.
- The probability score is calculated using softmax, which converts the raw outputs from the model into probabilities.
Testing the JSON Response
You can test this API using tools like Postman, cURL, or by creating a simple frontend application that interacts with the /predict endpoint.
Example using cURL:
curl -X POST -F “image=@image.jpg” http://127.0.0.1:5000/predict
Expected Response:
{
“prediction”: 5,
“probability”: 0.8765,
“message”: “Prediction successful”
}
Handling Errors in JSON Response
It’s important to handle errors properly and provide meaningful responses. For example, if the input image is missing or corrupted, you should return a proper error message in JSON format.
Here’s an example of an error response:
{
“error”: “Invalid image format”,
“message”: “The provided image is not in a valid format”
}
This ensures that clients can handle errors gracefully and take corrective action.
Frequently Asked Questions
- What other data formats can Flask serve apart from JSON?
- Answer: Flask can serve data in various formats, including XML, HTML, plain text, and CSV. However, JSON is the most commonly used format for machine learning APIs due to its ease of use and support across platforms.
- How can I change the response format to something other than JSON?
- Answer: You can return other formats by setting the Content-Type header. For example, to return an HTML page, use return render_template(‘page.html’). For plain text, use return “Hello, World!” with return Response(“message”, mimetype=’text/plain’).
- Can I add additional information in the JSON response, such as execution time?
- Answer: Yes, you can add any additional metadata in the JSON response. For example, you could include the time it took for the model to make a prediction or any other relevant details:
{
“prediction”: 5,
“probability”: 0.8765,
“execution_time”: “0.05s”,
“message”: “Prediction successful”
}
- How do I handle large JSON responses?
- Answer: If the response data is large, you can consider compressing the response or sending data in chunks. In Flask, you can enable gzip compression to reduce the size of large JSON responses:
from flask_compress import Compress
app = Flask(__name__)
Compress(app)
- Can I serve JSON from a database in the same way?
- Answer: Yes, you can serve data stored in a database in JSON format. You would query the database, format the results as a dictionary or list, and then use jsonify() to return it as JSON:
results = {“data”: database_query_result}
return jsonify(results)
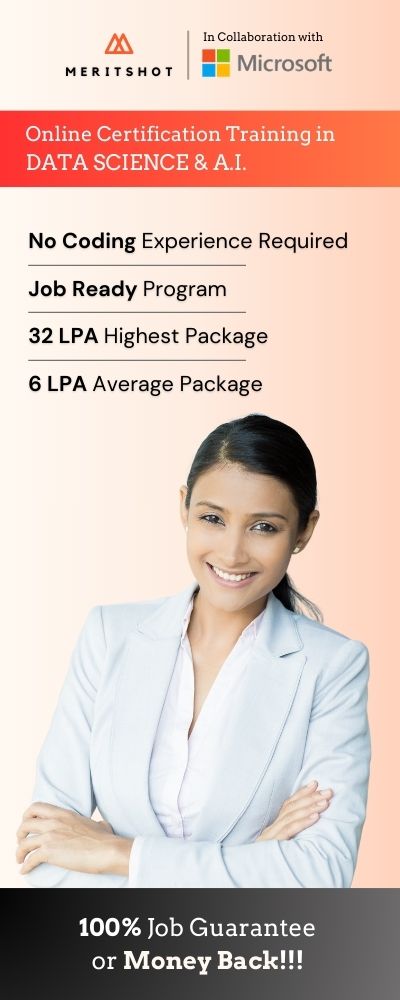